Toolbar customization and export settings in FastReport.Web for Core
September 6, 2021 by Oleg Kojnikov
Our users often need to change the appearance of the toolbar or customize the export menu, but not everyone knows how to do this. Let’s say that we already have a finished project. As an example, we can use any report from the FastReport .NET demo application.
Let’s add some colors to our toolbar. We need to write a code that will be responsible for customization:
Now let's run our application and see the result:
Для просмотра ссылки Войдиили Зарегистрируйся
Let’s take a look at how customization of the toolbar works in FastReport Web for Core in more detail.
All customization parameters are stored as a collection of properties. There are several options of how you can implement changes in the appearance of the toolbar, but they all come down to adding or changing parameters.
Let’s consider the appearance customization from the code, where you can see a list of collections and properties. Here are some of them:
To change the appearance of the dropdown menu, you just need to write some changes in the toolbar. But to display all the exporting data options, you need to add the following piece of code:
If we run our project, we will see that the dropdown menu has changed, and the exporting data options have significantly increased:
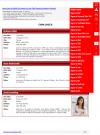
Now we see a customized menu with export formats.
But what if we need only certain formats? For example, we need PDF, XPS, and CSV only. Let’s implement it!
We need to slightly change the export settings in the container:
Let’s run our application and see the result:
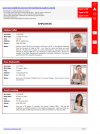
If only these export options are displayed, then you did everything right.
So, we have described how to customize the toolbar and edit the dropdown menu with export options in FastReport Web for Core. In addition to these examples, you can use the parameters discussed in combination with the other ones.
September 6, 2021 by Oleg Kojnikov
Our users often need to change the appearance of the toolbar or customize the export menu, but not everyone knows how to do this. Let’s say that we already have a finished project. As an example, we can use any report from the FastReport .NET demo application.
Let’s add some colors to our toolbar. We need to write a code that will be responsible for customization:
Код:
ToolbarSettings toolbar = new ToolbarSettings()
{
Color = Color.Red,
DropDownMenuColor = Color.IndianRed,
IconColor = IconColors.Left,
Position = Positions.Left,
IconTransparency = IconTransparencyEnum.Low,
};
webReport.Toolbar = toolbar;
Для просмотра ссылки Войди
Let’s take a look at how customization of the toolbar works in FastReport Web for Core in more detail.
All customization parameters are stored as a collection of properties. There are several options of how you can implement changes in the appearance of the toolbar, but they all come down to adding or changing parameters.
Let’s consider the appearance customization from the code, where you can see a list of collections and properties. Here are some of them:
- Color – change the background color of the toolbar.
- DropDownMenuColor – set the background color of the dropdown menu.
- DropDownMenuTextColor – set the text color of the dropdown menu.
- Position – change the toolbar position in the report.
- Roundness – add roundness to the toolbar.
- ContentPosition – change the content position.
- IconColor – change the icon colors.
- IconTransparency – adjust the icons transparency.
- FontSettings – fine-tune text styles.
To change the appearance of the dropdown menu, you just need to write some changes in the toolbar. But to display all the exporting data options, you need to add the following piece of code:
Код:
ToolbarSettings toolbar = new ToolbarSettings()
{
Color = Color.Red,
DropDownMenuColor = Color.Red,
DropDownMenuTextColor = Color.White,
IconColor = IconColors.White,
Position = Positions.Right,
FontSettings = new Font("Arial", 14, FontStyle.Bold),
Exports = new ExportMenuSettings()
{
ExportTypes = Exports.All
}
};
model.WebReport.Toolbar = toolbar;
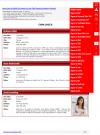
Now we see a customized menu with export formats.
But what if we need only certain formats? For example, we need PDF, XPS, and CSV only. Let’s implement it!
We need to slightly change the export settings in the container:
Код:
Exports = new ExportMenuSettings()
{
ExportTypes = Exports.Pdf | Exports.Xps | Exports.Csv
}
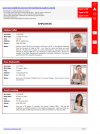
If only these export options are displayed, then you did everything right.
So, we have described how to customize the toolbar and edit the dropdown menu with export options in FastReport Web for Core. In addition to these examples, you can use the parameters discussed in combination with the other ones.