Learn To Simulate Realistic 2D Ball Physics in C++ Builder
By Yilmaz Yoru July 18, 2021
In this post we will explain how we can simulate realistic 2D ball physics in an easy and accessible way. Let’s assume that we look from a X-Y view, and Y is the height that means Y=0 is the ground. How we can simulate a ball physics in a given ball velocity, and gravity in that environment.
Для просмотра ссылки Войдиили Зарегистрируйся is a great compiler and IDE with FireMonkey and VCL frameworks. It has compilers for Win32, Win64, Android and iOS. C++Builder has both CLANG Enhanced C/C++ Compiler and a Borland C/C++ Compiler. It also features a modern, high-productivity RAD Studio IDE, debugger tools, and enterprise connectivity for to accelerate cross-platform UI development. We can develop GUI based applications easily, as it comes with the award-winning VCL framework for high-performance native Windows apps and the powerful FireMonkey (FMX) framework for cross-platform UIs. There is a freeДля просмотра ссылки Войди или Зарегистрируйся that can be used by students, beginners and startups with limitations.
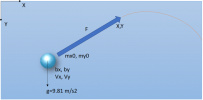
You can download Free the C++ Builder Community Edition (CE) here: Для просмотра ссылки Войдиили Зарегистрируйся.
Professional developers can use the Professional, Architect or Enterprise versions of C++ Builder. You can download and use the trial version for one month with the same capabilities as the full version . Please visit Для просмотра ссылки Войдиили Зарегистрируйся.
Let’s define balls
We can set all balls in our form creation as below;
For example, we can check all balls physics in a Timer as below,
Note that you can add rotation to image by changing its rotation. You can also touch it so user can throw this ball. You can develop many creative new games with C++ Builder as given example above.
By Yilmaz Yoru July 18, 2021
In this post we will explain how we can simulate realistic 2D ball physics in an easy and accessible way. Let’s assume that we look from a X-Y view, and Y is the height that means Y=0 is the ground. How we can simulate a ball physics in a given ball velocity, and gravity in that environment.
Для просмотра ссылки Войди
Understanding how 2D physics works
Let’s assume that we have a ball at bx and by coordinate with a given Vx and Vy velocity. Now we want to see what happens in every milliseconds under the gravitational environment.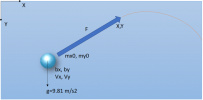
You can download Free the C++ Builder Community Edition (CE) here: Для просмотра ссылки Войди
Professional developers can use the Professional, Architect or Enterprise versions of C++ Builder. You can download and use the trial version for one month with the same capabilities as the full version . Please visit Для просмотра ссылки Войди
Let’s create a C++ class to simulate a ball
To simulate ball, we can create
C++:
class Tball
{
public:
TImage *image;
float x,y, vx,vy, ax, ay;
int W,H;
Tball() // Constructor
{
image = new TImage(Form1);
};
~Tball() //Deconstructor
{
image->Free();
}
};
C++:
#define NUMBER_OF_BALLS 10
Tball ball[NUMBER_OF_BALLS];
unsigned long int mseconds=0;
float g = 9.81;
C++:
__fastcall TForm1::TForm1(TComponent* Owner) : TForm(Owner)
{
srand(time(0));
for(int i=0; i<NUMBER_OF_BALLS; i++)
{
ball[i].image->Bitmap->Assign(ball_image1->Bitmap);
ball[i].image->Width =ball_image1->Width;
ball[i].image->Height =ball_image1->Height;
ball[i].image->Visible = true;
ball[i].x = rand()%800;
ball[i].y = rand()%800;
ball[i].vx = (rand()%100)/10.0;
ball[i].vy = (rand()%100)/10.0;
}
}
Applying the physics
Now we should apply physics in a Timer, we should check if our ball is touching to borders, touching the ground. We should change its velocity direction by multiplying with -1. We can also add some friction (velocity loss) so we can multiply with -0.98. Finally we should change its x y position with well known physics (V = V+g*t or X =Vt+gt*t etc.). We should apply all these in a timer. We can check many balls in a for loop.For example, we can check all balls physics in a Timer as below,
C++:
void __fastcall TForm1::Timer1Timer(TObject *Sender)
{
mseconds++;
float t=mseconds/1000;
for(int i=0; i<NUMBER_OF_BALLS; i++)
{
if(ball[i].y>Rectangle1->Height-ball[i].H-ball[i].vy) { ball[i].vy=fabs(ball[i].vy)*(-0.8); ball[i].vx*=0.98; }
if(ball[i].x>Rectangle1->Width- ball[i].W-ball[i].vx) { ball[i].vx=fabs(ball[i].vx)*(-0.8); }
if(ball[i].x<ball[i].vx) { ball[i].vx=fabs(ball[i].vx)*(0.8); }
ball[i].vy=ball[i].vy+g*0.06;
ball[i].x += ball[i].vx;
ball[i].y += ball[i].vy;
ball[i].x=Min((float)Rectangle1->Width-ball[i].W, ball[i].x+ball[i].vx);
ball[i].y=Min((float)Rectangle1->Height-ball[i].H, ball[i].y+ball[i].vy);
ball[i].image->Position->X=ball[i].x;
ball[i].image->Position->Y=ball[i].y;
}
}
Simulating ball movement every millisecond in C++
Timer to simulate every millisecond,
C++:
void __fastcall TForm1::Timer1Timer(TObject *Sender)
{
mseconds++;
float t=mseconds/1000;
for(int i=0; i<NUMBER_OF_BALLS; i++)
{
if(ball[i].y>Rectangle1->Height-ball[i].H-ball[i].vy) { ball[i].vy=fabs(ball[i].vy)*(-0.8); ball[i].vx*=0.98; }
if(ball[i].x>Rectangle1->Width- ball[i].W-ball[i].vx) { ball[i].vx=fabs(ball[i].vx)*(-0.8); }
if(ball[i].x<ball[i].vx) { ball[i].vx=fabs(ball[i].vx)*(0.8); }
ball[i].vy=ball[i].vy+g*0.06;
ball[i].x += ball[i].vx;
ball[i].y += ball[i].vy;
ball[i].x=Min((float)Rectangle1->Width-ball[i].W, ball[i].x+ball[i].vx);
ball[i].y=Min((float)Rectangle1->Height-ball[i].H, ball[i].y+ball[i].vy);
ball[i].image->Position->X=ball[i].x;
ball[i].image->Position->Y=ball[i].y;
}
}