[SHOWTOGROUPS=4,20,22]
Let's comment this function in module definition file:
Hide Copy Code
LIBRARY DLC
EXPORTS
_BinkSetPan@12 = binkw32._BinkSetPan@12
_BinkSetVolume@12 = binkw32._BinkSetVolume@12
_BinkGetError@0 = binkw32._BinkGetError@0
_BinkPause@8 = binkw32._BinkPause@8
_BinkOpen@8 = binkw32._BinkOpen@8
_BinkSetIO@4 = binkw32._BinkSetIO@4
_BinkSetSoundTrack@8 = binkw32._BinkSetSoundTrack@8
_BinkSetSoundSystem@8 = binkw32._BinkSetSoundSystem@8
_BinkOpenDirectSound@4 = binkw32._BinkOpenDirectSound@4
; _RADSetMemory@8 = binkw32._RADSetMemory@8
_BinkClose@4 = binkw32._BinkClose@4
_BinkNextFrame@4 = binkw32._BinkNextFrame@4
_BinkCopyToBufferRect@44 = binkw32._BinkCopyToBufferRect@44
_BinkDoFrame@4 = binkw32._BinkDoFrame@4
_BinkWait@4 = binkw32._BinkWait@4
_RADTimerRead@0 = binkw32._RADTimerRead@0
And provide our own implementation:
Hide Copy Code
typedef void *type_SomeFunctionPointer;
typedef void(__stdcall *type_binkw32_RADSetMemory)
(type_SomeFunctionPointer, type_SomeFunctionPointer);
type_binkw32_RADSetMemory binkw32_RADSetMemory;
void DLCInit()
{
...
HMODULE binkw32 = LoadLibraryA("binkw32.dll");
binkw32_RADSetMemory =
(type_binkw32_RADSetMemory)GetProcAddress(binkw32, "_RADSetMemory@8");
}
extern "C"
{
__declspec(dllexport) void __stdcall RADSetMemory
(type_SomeFunctionPointer f1, type_SomeFunctionPointer f2)
{
binkw32_RADSetMemory(f1, f2);
}
}
Let's build our dlc DLL with this small change, and verify its exports:
dumpbin /exports dlc.dll
We can debug it like any other code:
Why we bother to provide such stubs? This way, we can have reversed API interface expressed as C code, and we will immediately know if we didn't get it right (program will probably crash), because this function gets called actually. We can patch multiple import descriptors with our dlc DLL name, and either forward API to appropriate DLL, or provide our own stub as we advance through reverse process.
Now let's show 64-bit example. It will be port of doom3 source code called dhewm3:
[/SHOWTOGROUPS]
Let's comment this function in module definition file:
Hide Copy Code
LIBRARY DLC
EXPORTS
_BinkSetPan@12 = binkw32._BinkSetPan@12
_BinkSetVolume@12 = binkw32._BinkSetVolume@12
_BinkGetError@0 = binkw32._BinkGetError@0
_BinkPause@8 = binkw32._BinkPause@8
_BinkOpen@8 = binkw32._BinkOpen@8
_BinkSetIO@4 = binkw32._BinkSetIO@4
_BinkSetSoundTrack@8 = binkw32._BinkSetSoundTrack@8
_BinkSetSoundSystem@8 = binkw32._BinkSetSoundSystem@8
_BinkOpenDirectSound@4 = binkw32._BinkOpenDirectSound@4
; _RADSetMemory@8 = binkw32._RADSetMemory@8
_BinkClose@4 = binkw32._BinkClose@4
_BinkNextFrame@4 = binkw32._BinkNextFrame@4
_BinkCopyToBufferRect@44 = binkw32._BinkCopyToBufferRect@44
_BinkDoFrame@4 = binkw32._BinkDoFrame@4
_BinkWait@4 = binkw32._BinkWait@4
_RADTimerRead@0 = binkw32._RADTimerRead@0
And provide our own implementation:
Hide Copy Code
typedef void *type_SomeFunctionPointer;
typedef void(__stdcall *type_binkw32_RADSetMemory)
(type_SomeFunctionPointer, type_SomeFunctionPointer);
type_binkw32_RADSetMemory binkw32_RADSetMemory;
void DLCInit()
{
...
HMODULE binkw32 = LoadLibraryA("binkw32.dll");
binkw32_RADSetMemory =
(type_binkw32_RADSetMemory)GetProcAddress(binkw32, "_RADSetMemory@8");
}
extern "C"
{
__declspec(dllexport) void __stdcall RADSetMemory
(type_SomeFunctionPointer f1, type_SomeFunctionPointer f2)
{
binkw32_RADSetMemory(f1, f2);
}
}
Let's build our dlc DLL with this small change, and verify its exports:
dumpbin /exports dlc.dll
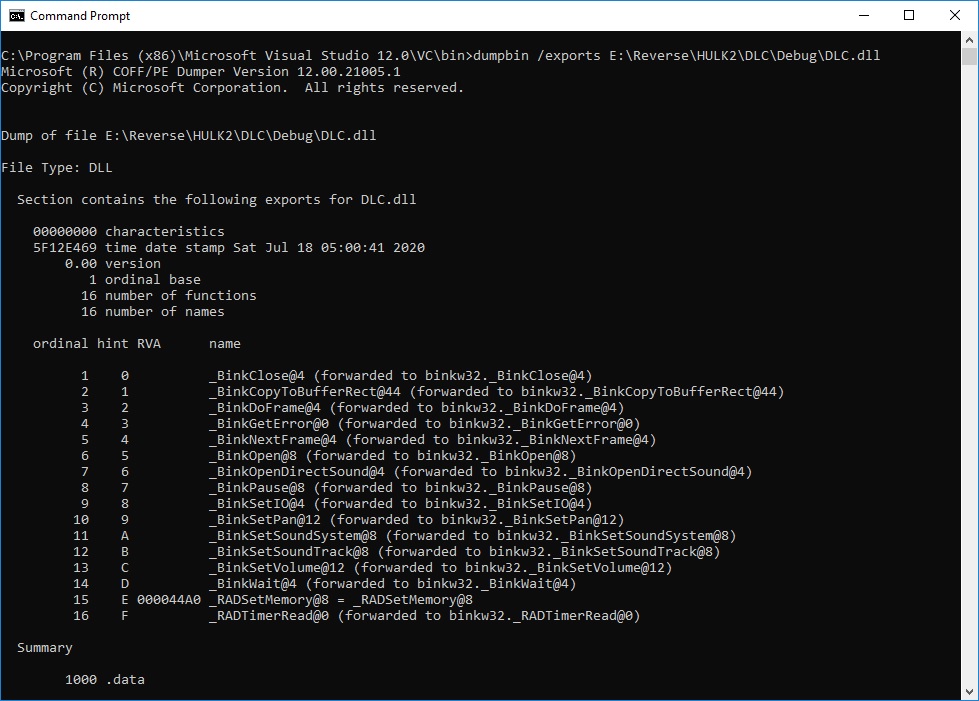
We can debug it like any other code:
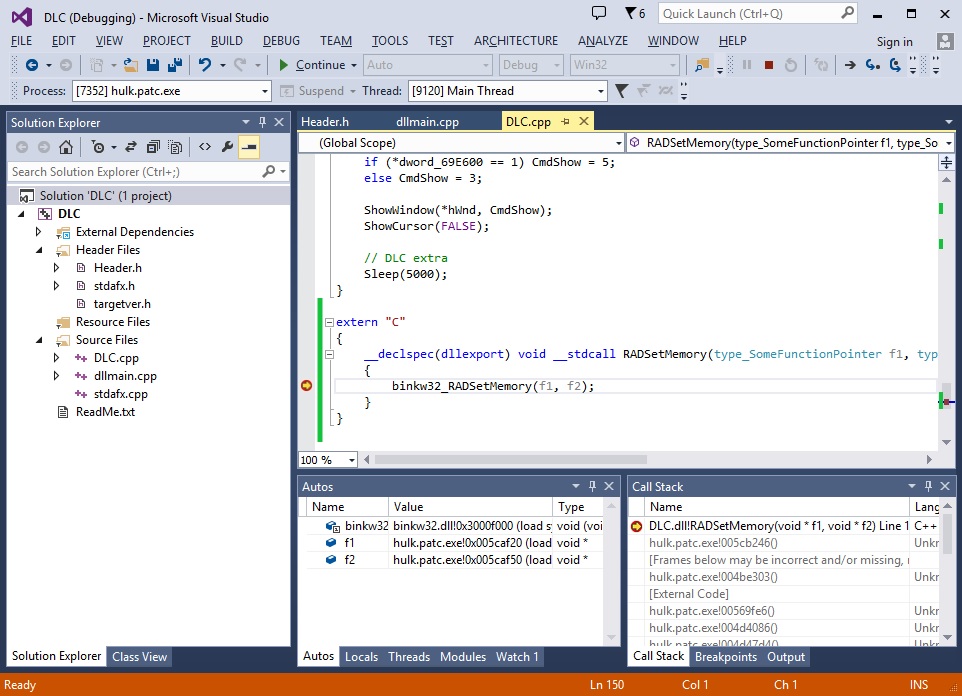
Why we bother to provide such stubs? This way, we can have reversed API interface expressed as C code, and we will immediately know if we didn't get it right (program will probably crash), because this function gets called actually. We can patch multiple import descriptors with our dlc DLL name, and either forward API to appropriate DLL, or provide our own stub as we advance through reverse process.
Now let's show 64-bit example. It will be port of doom3 source code called dhewm3:
- Для просмотра ссылки Войди
или Зарегистрируйся
- Для просмотра ссылки Войди
или Зарегистрируйся
[/SHOWTOGROUPS]