Working with big data databases in Delphi – Cassandra, Couchbase and MongoDB (Part 2 of 3)
January 11, 2017 Allen Drennan
January 11, 2017 Allen Drennan
[SHOWTOGROUPS=4,20]
This is the second part of a three-part series on working with big data databases directly from Delphi. In this second part we focus on a basic class framework for working with Couchbase along with unit tests and examples.
Для просмотра ссылки Войдиили Зарегистрируйся focuses on Cassandra, Для просмотра ссылки Войди или Зарегистрируйся focuses on Couchbase and Part 3 focuses on MongoDB.
For more information about us, our support and services visit the Для просмотра ссылки Войдиили Зарегистрируйся or the Для просмотра ссылки Войди или Зарегистрируйся.
The example contained here depends upon part of our Для просмотра ссылки Войдиили Зарегистрируйся.
The source code and related example repository is hosted on GitHub at Для просмотра ссылки Войдиили Зарегистрируйся.
Introduction to Couchbase
Для просмотра ссылки Войдиили Зарегистрируйся is a high-performance NoSQL database renowned for its speed and flexibility.
It combines some of the best aspects of other leading NoSQL databases into a model which is easy to deploy, maintain and scale. Couchbase offers many things in addition to administrative tools, which are included, they also have solutions for mobile platforms. Couchbase is widely used in Internet and cloud based apps and services and continues to grow in popularity.
Couchbase isn’t directly tied to storing and retrieving JSON, any binary content can be stored and easily retrieved. However, Couchbase offers subdocument capabilities that provide direct JSON manipulation (much like MongoDB) but doesn’t directly tie you to a rigid schema.
This is by no means an exhaustive look at the benefits of Couchbase. If you are truly interested there are wealth of Для просмотра ссылки Войдиили Зарегистрируйся.
Delphi and Couchbase
In order to use Couchbase from Delphi we created a header conversion for the latest C library SDK interface provided by Couchbase. The C/C++ interface is relatively new, and provides the most common CRUD operations but also provides subdocument APIs for working directly with JSON documents.
The examples here is for Delphi on Windows using a Couchbase remote database running on Linux. We use Для просмотра ссылки Войдиили Зарегистрируйся for our examples.
Installing Couchbase Server
Installing the Couchbase server is a fairly straightforward effort thanks to excellent documentation available on their website. We prefer Ubuntu so the instructions set forth here are focused on that Linux flavor.
Installing Couchbase involves 3 main steps:
On Ubuntu if you want Couchbase to always start when the system restarts, then type:
LibCouchbase
In order to interact with Couchbase from Delphi we use the C/C++ SDK library provided by Couchbase. The library headers are converted to Delphi and we wrap it in an easy to use Delphi class architecture.
The Для просмотра ссылки Войдиили Зарегистрируйся for Couchbase can be located under the C SDK section of the website.
For our purposes we are using the libcouchbase.dll library contained in the Visual Studio 2012 x86-vc11 library.
TgoCouchbase Class Architecture
The TgoCouchbase class architecture closely mirrors the API model provided by Couchbase for other APIs such as C/C++ and Python with most of the core operations named the same.
Connecting to Couchbase
To connect to Couchbase you first create an instance of the TgoCouchbase class and then call Connect using a Couchbase connection string which in our case is the address of your Couchbase server.
If the connection fails you can examine Couchbase.LastErrorCode and Couchbase.LastErrorDesc. A list of error codes are provided below.
[/SHOWTOGROUPS]
This is the second part of a three-part series on working with big data databases directly from Delphi. In this second part we focus on a basic class framework for working with Couchbase along with unit tests and examples.
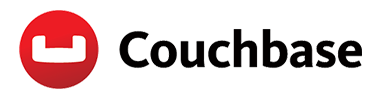
Для просмотра ссылки Войди
For more information about us, our support and services visit the Для просмотра ссылки Войди
The example contained here depends upon part of our Для просмотра ссылки Войди
The source code and related example repository is hosted on GitHub at Для просмотра ссылки Войди
Introduction to Couchbase
Для просмотра ссылки Войди
It combines some of the best aspects of other leading NoSQL databases into a model which is easy to deploy, maintain and scale. Couchbase offers many things in addition to administrative tools, which are included, they also have solutions for mobile platforms. Couchbase is widely used in Internet and cloud based apps and services and continues to grow in popularity.
Couchbase isn’t directly tied to storing and retrieving JSON, any binary content can be stored and easily retrieved. However, Couchbase offers subdocument capabilities that provide direct JSON manipulation (much like MongoDB) but doesn’t directly tie you to a rigid schema.
This is by no means an exhaustive look at the benefits of Couchbase. If you are truly interested there are wealth of Для просмотра ссылки Войди
Delphi and Couchbase
In order to use Couchbase from Delphi we created a header conversion for the latest C library SDK interface provided by Couchbase. The C/C++ interface is relatively new, and provides the most common CRUD operations but also provides subdocument APIs for working directly with JSON documents.
The examples here is for Delphi on Windows using a Couchbase remote database running on Linux. We use Для просмотра ссылки Войди
Installing Couchbase Server
Installing the Couchbase server is a fairly straightforward effort thanks to excellent documentation available on their website. We prefer Ubuntu so the instructions set forth here are focused on that Linux flavor.
Installing Couchbase involves 3 main steps:
- Install Для просмотра ссылки Войди
или Зарегистрируйся. - Для просмотра ссылки Войди
или Зарегистрируйся for Ubuntu (currently 14.04 official) - Install Couchbase Server (currently 4.6 pre-release)
1 | sudo dpkg -i couchbase-server-enterprise_4.6.0-DP-ubuntu14.04_amd64.deb |
1 2 | systemctl start couchbase-server systemctl enable couchbase-server |
In order to interact with Couchbase from Delphi we use the C/C++ SDK library provided by Couchbase. The library headers are converted to Delphi and we wrap it in an easy to use Delphi class architecture.
The Для просмотра ссылки Войди
For our purposes we are using the libcouchbase.dll library contained in the Visual Studio 2012 x86-vc11 library.
TgoCouchbase Class Architecture
The TgoCouchbase class architecture closely mirrors the API model provided by Couchbase for other APIs such as C/C++ and Python with most of the core operations named the same.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | TgoCouchbase = class(TObject) public constructor Create; destructor Destroy; override; public function Connect(const AConnection: String; const AUsername: String = ''; const APassword: String = ''): Boolean; { Get } function Get(const AKey: Utf8String; out AValue: TBytes): TCouchbaseResult; overload; function Get(const AKey: Utf8String; out AValue: String): TCouchbaseResult; overload; { Set/Upsert } function Upsert(const AKey: Utf8String; const AValue: TBytes; const AOptions: TCouchbaseOptions): TCouchbaseResult; overload; function Upsert(const AKey: Utf8String; const AValue: String; const AOptions: TCouchbaseOptions): TCouchbaseResult; overload; function Upsert(const AKey: Utf8String; const AValue: String): TCouchbaseResult; overload; { Add } function Add(const AKey: Utf8String; const AValue: TBytes; const AOptions: TCouchbaseOptions): TCouchbaseResult; overload; function Add(const AKey: Utf8String; const AValue: String; const AOptions: TCouchbaseOptions): TCouchbaseResult; overload; function Add(const AKey: Utf8String; const AValue: String): TCouchbaseResult; overload; { Replace } function Replace(const AKey: Utf8String; const AValue: TBytes; const AOptions: TCouchbaseOptions): TCouchbaseResult; overload; function Replace(const AKey: Utf8String; const AValue: String; const AOptions: TCouchbaseOptions): TCouchbaseResult; overload; function Replace(const AKey: Utf8String; const AValue: String): TCouchbaseResult; overload; { Append/Prepend } function Append(const AKey: Utf8String; const AValue: TBytes): TCouchbaseResult; overload; function Append(const AKey: Utf8String; const AValue: String): TCouchbaseResult; overload; function Prepend(const AKey: Utf8String; const AValue: TBytes): TCouchbaseResult; overload; function Prepend(const AKey: Utf8String; const AValue: String): TCouchbaseResult; overload; { Touch } function Touch(const AKey: Utf8String; const AExpireTime: UInt32 = 0): TCouchbaseResult; { Increment/Decrement } function Incr(const AKey: Utf8String; const ADelta: Integer = 1; const AInitial: Integer = 0; const ACreate: Boolean = True): TCouchbaseResult; function Decr(const AKey: Utf8String; const ADelta: Integer = -1; const AInitial: Integer = 0; const ACreate: Boolean = True): TCouchbaseResult; { Delete } function Delete(const AKey: Utf8String): TCouchbaseResult; { Flush } function Flush: TCouchbaseFlushResult; { Stats } function Stats: TCouchbaseStatsResult; public { Subdocuments } { Lookup subdoc operations } function LookupIn(const AKey: Utf8String): ICouchbaseSubDoc; { Mutate subdoc operations } function MutateIn(const AKey: Utf8String): ICouchbaseSubDoc; public { N1QL } function Query(const AParams: TgoCouchbaseN1QL): TCouchbaseQueryResult; public property LastErrorCode: Integer read FLastErrorCode; property LastErrorDesc: String read FLastErrorDesc; { Bucket instance } property Instance: lcb_t read FInstance; end; |
To connect to Couchbase you first create an instance of the TgoCouchbase class and then call Connect using a Couchbase connection string which in our case is the address of your Couchbase server.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | var Couchbase := TgoCouchbase.Create; ReturnValue: Boolean; begin Couchbase := TgoCouchbase.Create; try ReturnValue := Couchbase.Connect('couchbase://192.168.1.84'); if ReturnValue then begin // Connected end else Writeln(Couchbase.LastErrorDesc); finally Couchbase.Free; end; end; |
[/SHOWTOGROUPS]