Secure External APIs with API Keys - and Keep the Villains at Bay
Lee P. Richardson - 26/Jun/2020
Lee P. Richardson - 26/Jun/2020
[SHOWTOGROUPS=4,20]
How to secure external web API's?
This blog post is about how to secure external web API's, as shown with diagrams, detailed videos, and a whole working Pull Request in a sample GitHub repo. This post is fairly specific to the security model in the ASP.NET Boilerplate framework, but even if you're not using that framework, this technique should be generally applicable.
Suppose you've written a web app and exposed an external REST API based on Swagger and Swashbuckle in an ASP.NET Core site. Perhaps you blindly followed some rando's recent Для просмотра ссылки Войдиили Зарегистрируйся about it, published it to production, and your customers are super happy except: Horrors, you've just realized you forgot to secure it and now it's open to all the villains of the Internet! Worse, your customers don't want to use their existing credentials, they want something called API Keys.
Good news: you've just stumbled on said Для просмотра ссылки Войдиили Зарегистрируйся's follow-up blog post about how to secure those external web API's, and even better it's got diagrams, detailed videos, and a whole working Для просмотра ссылки Войди или Зарегистрируйся in a sample GitHub repo.
One caveat: this post is fairly specific to the security model in the Для просмотра ссылки Войдиили Зарегистрируйся framework, but even if you're not using that framework, this technique should be generally applicable.
What Are API Keys?
The idea of API Keys is fairly standard with systems that offer an external API. They offer customers a way to connect to an API with credentials that are separate from their own. More importantly API Keys offer limited access, a critical element of a good security model. API Keys can't, for instance, log in to the site and create new API Keys, or manage users. They can only perform the limited actions that the external Web API grants. If the credentials become compromised, users can reject or delete them without affecting their own credentials.
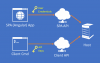
Data Structure
The first step in implementing API Keys is to design the data structure and build out the CRUD for the UI. Well, the CRD, anyway, updating API Keys doesn't make much sense.
Conveniently enough ASP.NET Boilerplate already provides a Users entity that ostensibly offers a superset of everything required. ASP.NET Boilerplate Users can have multiple Roles and each of those Roles can have multiple Permissions.
Developers can then restrict access to methods and classes via the AbpAuthorize("SomePermission") attribute, which takes a Permission as a parameter. When an end user makes a request to an endpoint ABP determines the user from a bearer token in the request header, figures out their roles, and figures out which permissions belong to those roles. If one of those permissions matches the requirement in the AbpAuthorize() attribute, the call is allowed through.
API Keys should be similar. As far as fields they'll have an "API Key" instead of "Username", and a "Secret" instead of a "Password". Unlike users they'll likely only need one permission for decorating the external API instead of many. Thus, they'll have just a single Role to help link the single permission to the API Keys.
Therefore, implementing this should be as simple as having ApiKey inherit from User and pretend that Username is ApiKey and Password is Secret.
Crudy Keys
Last month this rando also published an Для просмотра ссылки Войдиили Зарегистрируйся to help build out CRUD for new entities in ASP.NET Boilerplate apps. Following that guide for creating API Keys would be a great place to start. I'll include the original step and any customizations below. Also, if you want to follow along visually this dude also posted a video of the process including explaining topics like Table-Per-Hierarchy inheritance and published it as an episode to something called Для просмотра ссылки Войди или Зарегистрируйся (to which you should totally subscribe):
[/SHOWTOGROUPS]
How to secure external web API's?
This blog post is about how to secure external web API's, as shown with diagrams, detailed videos, and a whole working Pull Request in a sample GitHub repo. This post is fairly specific to the security model in the ASP.NET Boilerplate framework, but even if you're not using that framework, this technique should be generally applicable.
Suppose you've written a web app and exposed an external REST API based on Swagger and Swashbuckle in an ASP.NET Core site. Perhaps you blindly followed some rando's recent Для просмотра ссылки Войди
Good news: you've just stumbled on said Для просмотра ссылки Войди
One caveat: this post is fairly specific to the security model in the Для просмотра ссылки Войди
What Are API Keys?
The idea of API Keys is fairly standard with systems that offer an external API. They offer customers a way to connect to an API with credentials that are separate from their own. More importantly API Keys offer limited access, a critical element of a good security model. API Keys can't, for instance, log in to the site and create new API Keys, or manage users. They can only perform the limited actions that the external Web API grants. If the credentials become compromised, users can reject or delete them without affecting their own credentials.
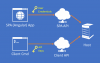
Data Structure
The first step in implementing API Keys is to design the data structure and build out the CRUD for the UI. Well, the CRD, anyway, updating API Keys doesn't make much sense.
Conveniently enough ASP.NET Boilerplate already provides a Users entity that ostensibly offers a superset of everything required. ASP.NET Boilerplate Users can have multiple Roles and each of those Roles can have multiple Permissions.

Developers can then restrict access to methods and classes via the AbpAuthorize("SomePermission") attribute, which takes a Permission as a parameter. When an end user makes a request to an endpoint ABP determines the user from a bearer token in the request header, figures out their roles, and figures out which permissions belong to those roles. If one of those permissions matches the requirement in the AbpAuthorize() attribute, the call is allowed through.
API Keys should be similar. As far as fields they'll have an "API Key" instead of "Username", and a "Secret" instead of a "Password". Unlike users they'll likely only need one permission for decorating the external API instead of many. Thus, they'll have just a single Role to help link the single permission to the API Keys.
Therefore, implementing this should be as simple as having ApiKey inherit from User and pretend that Username is ApiKey and Password is Secret.
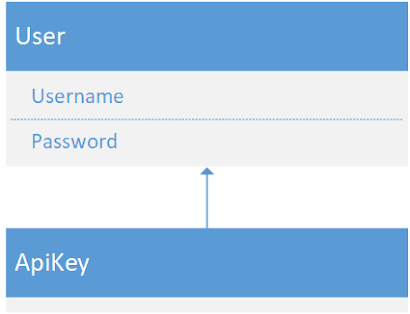
Crudy Keys
Last month this rando also published an Для просмотра ссылки Войди
[/SHOWTOGROUPS]