Reusable AngularJS Component: Warning Stripe by Han Bo Sun
Han Bo Sun - 03/Aug/2020
Han Bo Sun - 03/Aug/2020
[SHOWTOGROUPS=4,20,22]
Building a reusable component with AngularJS
In this tutorial, I will discuss how to build a reusable component with AngularJS, a status text display that can be used everywhere.
Introduction
One of the cool aspects of AngularJS are the directives. Directives can be created as customized components that can be placed on the HTML page. According to AngularJS documentation, directives are DOM element markers. When AngularJS applications runs, it compiles these markers, creates additional DOM elements as children to the marker, and attaches dynamic behaviors to it. This is a basic introduction of what a directive is.
Let's look at an example:
<select class="form-control" ng-model="vm.selectedValue"
ng-options="item as item.text for item of vm.optionValues">
</select>
This is a simple drop down list. Inside, there are two directives, one is ng-model, it can also be referred as ngModel. This directive is the most common one, it binds a view model property to the UI element. The other ng-options or ngOptions is another directive. It iterates through the data model, in this case, a list of items, and displays the items in the drop down control. These two are attributes based directives. For this tutorial, I will create a simple directive to demo how a typical directive can be created. It will be an element or attribute based directive.
Overview of the Sample Project
The sample project has just one page, with a user registration form. When user enters data on this page and something is missing, a warning stripe will be displayed on the top. To dismiss this warning stripe, user can click anyway in it, and it will disappear. When all required information has been entered, then user clicks on the button "Submit", the warning display will be replaced with a success message.
Here is a screenshot of the page with no user data entered:
Here is a screenshot of the page with error displayed:
When the user data is successfully submitted, here is a screenshot of the page with success message displayed:
The actual component that I want to share in this tutorial is this warning stripe. It is one of these little HTML pieces that can be placed anywhere in a web application. The code for such component can be duplicated everywhere, and most of the time, changing one of them does not have to be replicated at other places. But I had code duplication. Whenever there is an opportunity to create something reusable, I would try to create it. This tutorial will show how this little warning stripe is created, and how it can be used.
The sample project is Spring Boot based. The Java side of the project only to host static contents - the page that displays the user registration, as well as the JavaScript files, and other page files. I won't show any code from the Java side. If you are interested, please check them out when you have time.
Next, I will start discussing the design of the directive, which is all JavaScript and HTML.
The Design of the Warning Stripe Directive
The reason I call this directive a warning stripe is that in most of the cases, this directive will display errors or warnings. When it displays a warning or an error, the background color will be red. Occasionally, it can also be used to display success messages. When it displays success message, the background color would be a pleasing green. Yep. This directive is designed to display both types of messages.
I would like to show you how this directive is used in the main application. Then I can show how this directive is defined. In the file index.html, you will find something like this:
<div info-display op-success="vm.opSuccess" msg-to-show="vm.statusMessage" ></div>
Here, I added a div, and one attribute of this div is info-display. This info-display is the directive. When the application runs, AngularJS will compile and link this div with more HTML mark up. underneath it.
Notice the directive attribute is used as "info-display". This is an AngularJS convention. The directive is actually called infoDisplay. When you actually use it, the name is all lower case and words are connected by dash (or apostrophe). This is how AngularJS locates the directive from markup to the directive definition. Exactly how this is done I am not quite sure, but I do know the end result. Here is the markup of the alert stripe being displayed:
<div class="row ng-scope" ng-if="msgToShow != null && msgToShow.length > 0">
<div class="col-xs-12">
<div class="alert info-display ng-binding alert-success"
ng-class="{ 'alert-success': opSuccess, 'alert-danger': !opSuccess }"
ng-click="info.clickOnStatus()">
User registration request has been processed successfully.</div>
</div>
</div>
Obviously, some type of run-time HTML injection is happening here. This means I have to defined the HTML mark up of the innjected component. In the file called static/assets/app/pages/infoDisplay.html, you can find the mark up of such injection. Here it is:
<div class="row" ng-if="msgToShow != null && msgToShow.length > 0">
<div class="col-xs-12">
<div class="alert info-display"
ng-class="{ 'alert-success': opSuccess, 'alert-danger': !opSuccess }"
ng-click="info.clickOnStatus()">{{msgToShow}}</div>
</div>
</div>
This piece of markup is pretty simple, but there are a few points of interest that should be discussed. First, ngIf or ng-if directive. It is used as:
<div class="row" ng-if="msgToShow != null && msgToShow.length > 0">
...
</div>
[/SHOWTOGROUPS]
Building a reusable component with AngularJS
In this tutorial, I will discuss how to build a reusable component with AngularJS, a status text display that can be used everywhere.
Introduction
One of the cool aspects of AngularJS are the directives. Directives can be created as customized components that can be placed on the HTML page. According to AngularJS documentation, directives are DOM element markers. When AngularJS applications runs, it compiles these markers, creates additional DOM elements as children to the marker, and attaches dynamic behaviors to it. This is a basic introduction of what a directive is.
Let's look at an example:
<select class="form-control" ng-model="vm.selectedValue"
ng-options="item as item.text for item of vm.optionValues">
</select>
This is a simple drop down list. Inside, there are two directives, one is ng-model, it can also be referred as ngModel. This directive is the most common one, it binds a view model property to the UI element. The other ng-options or ngOptions is another directive. It iterates through the data model, in this case, a list of items, and displays the items in the drop down control. These two are attributes based directives. For this tutorial, I will create a simple directive to demo how a typical directive can be created. It will be an element or attribute based directive.
Overview of the Sample Project
The sample project has just one page, with a user registration form. When user enters data on this page and something is missing, a warning stripe will be displayed on the top. To dismiss this warning stripe, user can click anyway in it, and it will disappear. When all required information has been entered, then user clicks on the button "Submit", the warning display will be replaced with a success message.
Here is a screenshot of the page with no user data entered:
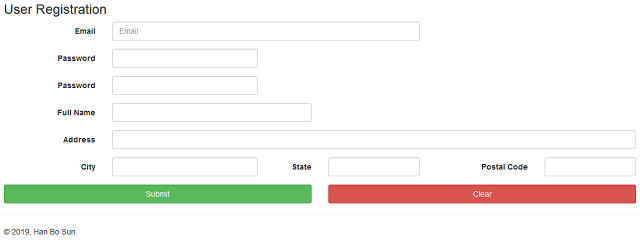
Here is a screenshot of the page with error displayed:
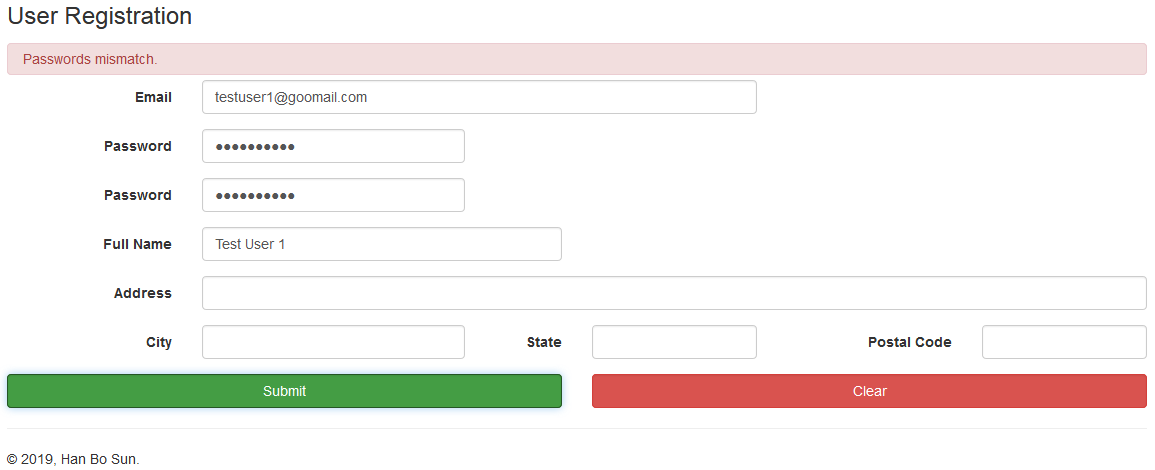
When the user data is successfully submitted, here is a screenshot of the page with success message displayed:
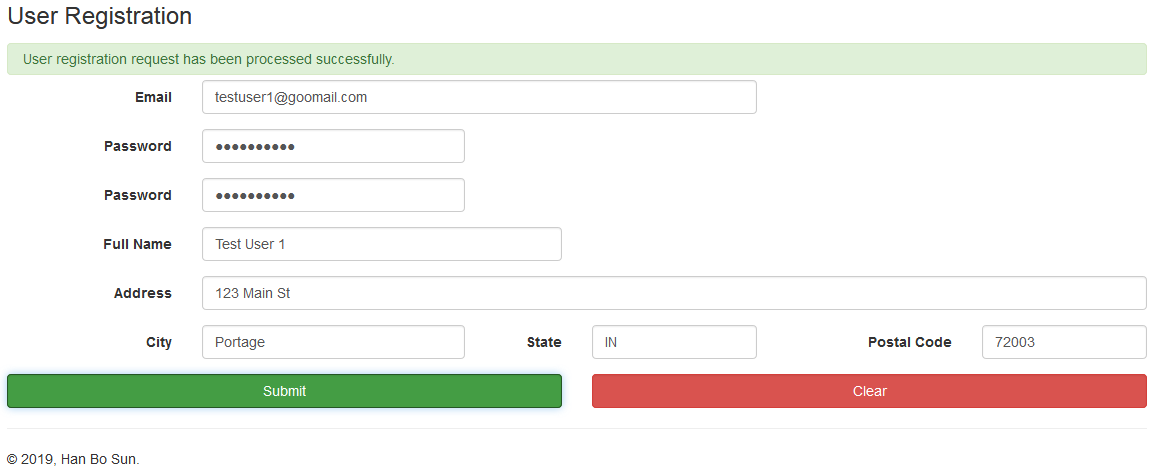
The actual component that I want to share in this tutorial is this warning stripe. It is one of these little HTML pieces that can be placed anywhere in a web application. The code for such component can be duplicated everywhere, and most of the time, changing one of them does not have to be replicated at other places. But I had code duplication. Whenever there is an opportunity to create something reusable, I would try to create it. This tutorial will show how this little warning stripe is created, and how it can be used.
The sample project is Spring Boot based. The Java side of the project only to host static contents - the page that displays the user registration, as well as the JavaScript files, and other page files. I won't show any code from the Java side. If you are interested, please check them out when you have time.
Next, I will start discussing the design of the directive, which is all JavaScript and HTML.
The Design of the Warning Stripe Directive
The reason I call this directive a warning stripe is that in most of the cases, this directive will display errors or warnings. When it displays a warning or an error, the background color will be red. Occasionally, it can also be used to display success messages. When it displays success message, the background color would be a pleasing green. Yep. This directive is designed to display both types of messages.
I would like to show you how this directive is used in the main application. Then I can show how this directive is defined. In the file index.html, you will find something like this:
<div info-display op-success="vm.opSuccess" msg-to-show="vm.statusMessage" ></div>
Here, I added a div, and one attribute of this div is info-display. This info-display is the directive. When the application runs, AngularJS will compile and link this div with more HTML mark up. underneath it.
Notice the directive attribute is used as "info-display". This is an AngularJS convention. The directive is actually called infoDisplay. When you actually use it, the name is all lower case and words are connected by dash (or apostrophe). This is how AngularJS locates the directive from markup to the directive definition. Exactly how this is done I am not quite sure, but I do know the end result. Here is the markup of the alert stripe being displayed:
<div class="row ng-scope" ng-if="msgToShow != null && msgToShow.length > 0">
<div class="col-xs-12">
<div class="alert info-display ng-binding alert-success"
ng-class="{ 'alert-success': opSuccess, 'alert-danger': !opSuccess }"
ng-click="info.clickOnStatus()">
User registration request has been processed successfully.</div>
</div>
</div>
Obviously, some type of run-time HTML injection is happening here. This means I have to defined the HTML mark up of the innjected component. In the file called static/assets/app/pages/infoDisplay.html, you can find the mark up of such injection. Here it is:
<div class="row" ng-if="msgToShow != null && msgToShow.length > 0">
<div class="col-xs-12">
<div class="alert info-display"
ng-class="{ 'alert-success': opSuccess, 'alert-danger': !opSuccess }"
ng-click="info.clickOnStatus()">{{msgToShow}}</div>
</div>
</div>
This piece of markup is pretty simple, but there are a few points of interest that should be discussed. First, ngIf or ng-if directive. It is used as:
<div class="row" ng-if="msgToShow != null && msgToShow.length > 0">
...
</div>
[/SHOWTOGROUPS]